Dictionary In Python
Published: June 18, 2022, 9:05 a.m.
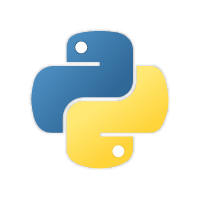
Introduction
In this article, I am going to teach you about the dictionary data structure python.
Creation
A dictionary is an ordered data structure in python. It holds key-value pairs. A dictionary is a python implementation of a “has a table”. You can access key-value pairs in constant time or O(1).
You can declare a dictionary like this
my_dic = {}
The {} tells the python interpreter that you are declaring a list
my_dic = dict()
You can use the built-in python dict() function to declare a dictionary.
#Syntax
my_dic = {key:value}
Let’s add some key-value pairs to your dictionary.
my_dic = {1: "cat", 2: "rat", 3:"mat", 4: "sat"}
Each item in our dictionary has to have a key along with a value, and we separate the items by using a comma.
Accessing An Item
Let's say we want to access the cat item from our dictionary, we would have to pass the key 1 in order to access the value cat.
print(my_dic[1]) # output βcatβ
We can access the other items in the same way
print(my_dic[2]) # output "rat"
print(my_dic[3]) # output "mat"
The key does not have to be an integer it can be any immutable datatype such as a string, tuple, float, and int, but you cannot use a list because it's a mutual datatype that can change.
Let's define a dictionary thats has keys with strings
person={"first_name": "Mark", "age":38, "job": "doctor"}
We can access each value using the same syntax as before but now we pass in a string as key
print(person["first_name"]) # output "mark"
print(person["age"]) # output 38
print(person["job"]) # output doctor
Adding Items to Dictionary
Let's say we want to add a last name to the person dictionary we created earlier. We would use the following syntax
Person["last_name"] = "Johnson"
If we print out the Person dictionary you will see last_name is added to the dictionary
print(person) #output {"first_name": "Mark",
# "age":38,
#"job": "doctor",
# Last_name: "Johnson"}
Overwriting values
Let's say we want to change last_name from “Johnson” to “Smith” we would write
Person["last_name"] = "Smith"
print(person) #output {"first_name": "Mark",
# "age":38,
#"job": "doctor",
# Last_name: "Smith"}
Removing Items From Dictionary
You can remove an element from a dictionary by using the .pop() method
If you call .pop on your person dictionary it will remove the last item in the dictionary
person={"first_name": "Mark", "age":38, "job": "doctor"}
person.pop() # remove last item
#output {"first_name": "Mark", "age":38}
The .pop() method can also take in a key of the item you want to remove.
#Syntax .pop(key)
person={"first_name": "Mark", "age":38}
person.pop("age") # remove last item
#output {"first_name": "Mark"}
Iterating over a dictionary
The first we to iterate over a dictionary is
for x in person:
print(x)
Second why to do this
for x in person.items():
print(x)
If we just want the keys from the dictionary
for x in person.keys():
print(x)
Or just want the values
for x in person.values():
print(x)
.key() method of dictionary returns a list of key so we can access a key by index.
my_keys = person.keys()
print(my_keys[0]) # output first_name
You do the same for values as well. The .value() method also returns a list
my_values = person.values()
print(my_values[0]) # output mark
Check If Value Is In Dictionary
If we want to check if a key exist in a dictionary we write the following code.
person={"first_name": "Mark", "age":38}
if "first_name" in person:
print("yes")
else
print("no")
#output yes
if "middle_name" in person:
print("yes")
else
print("no")
#output no